Fraud iOS SDK
A high-level guide for installing Forter's mobile SDK for native iOS Apps
This guide is for installing Forter's iOS SDK for native iOS applications! Note that you'll need a Forter portal account and your unique Forter credentials to complete an SDK integration. The steps below outline the high-level integration flow.
Step 1: Install the SDK 🍎
Forter's iOS SDK is distributed both on Swift Package Manager and CocoaPods.
Swift Package Manager
Xcode
- Navigate to File > Add Packages, paste Forter's distribution link (can be retrieved from the portal) to the search controller on the top right and press Add Package.
- Select the ForterSDK product and press Add Package
Package.swift
- Alternatively, if you have
Package.swift
dependencies file, you can add the SDK directly to the dependencies array.
dependencies: [
.package(url: "<forter-distribution-link>", .upToNextMajor(from: "3.0.2"))
]
- Then add ForterSDK to the dependencies array of the target:
targets: [
.target(
name: "MyTarget",
dependencies: [
.product(name: "ForterSDK", package: "forter-ios-sdk")
),
CocoaPods
- Make sure your your Podfile includes the
use_frameworks!
flag. - Add the ForterSDK pod to the Podfile next to the rest of pods used in your app:
pod 'ForterSDK', :git => <forter-distribution-link>'
- Run the
pod install
command
Step 2: Initiate the SDK ✨
Initialize your SDK from the iOS Application Delegate. This will ensure that it loads correctly when the app is active. For more information on the app delegate, please see the Official iOS Developer Documentation
- Import the SDK
import ForterSDK
#import <ForterSDK/ForterSDK.h>
- Setup the SDK on app launch using your siteId found in Forter portal for the appropriate environment, and a unique mobile device identifier. This identifier should be:
- Specific to each device
- Does not mutate from a user's app session
- PII compliant
Forter recommends using the iOS IDFV (Identifier For Vendor).
Casing While Forter's system has no casing preference, the
mobileUID
generated via the SDK must match the casing of the mobileUID that is passed to the Validation API at the time of transaction in order for Forter's system to correctly connect the SDK data to the backend, Validation API's data.
ForterSDK.setup(withDeviceUid:UIDevice.current.identifierForVendor.uuidString, siteId: "your_forter_site_id")
[ForterSDK setupWithDeviceUid:[[UIDevice currentDevice].identifierForVendor UUIDString] siteId:@"your_forter_site_id"];
- Add callbacks from your Application Delegate
- When receiving messages from your Application Delegate, you'll call specific Forter SDK methods when your iOS Application becomes active.
- 🔥 Please make sure to call ALL the AppDelegate callbacks 🔥
Step 3: Test/View your mobile events 📈
Once you've integrated the mobile SDK in sandbox (using your sandbox SiteId), you can look up events being passed to Forter for a specific mobileUID using our Sandbox Mobile Events Viewer. This gives developers visibility into how Forter is receiving and storing mobile events.
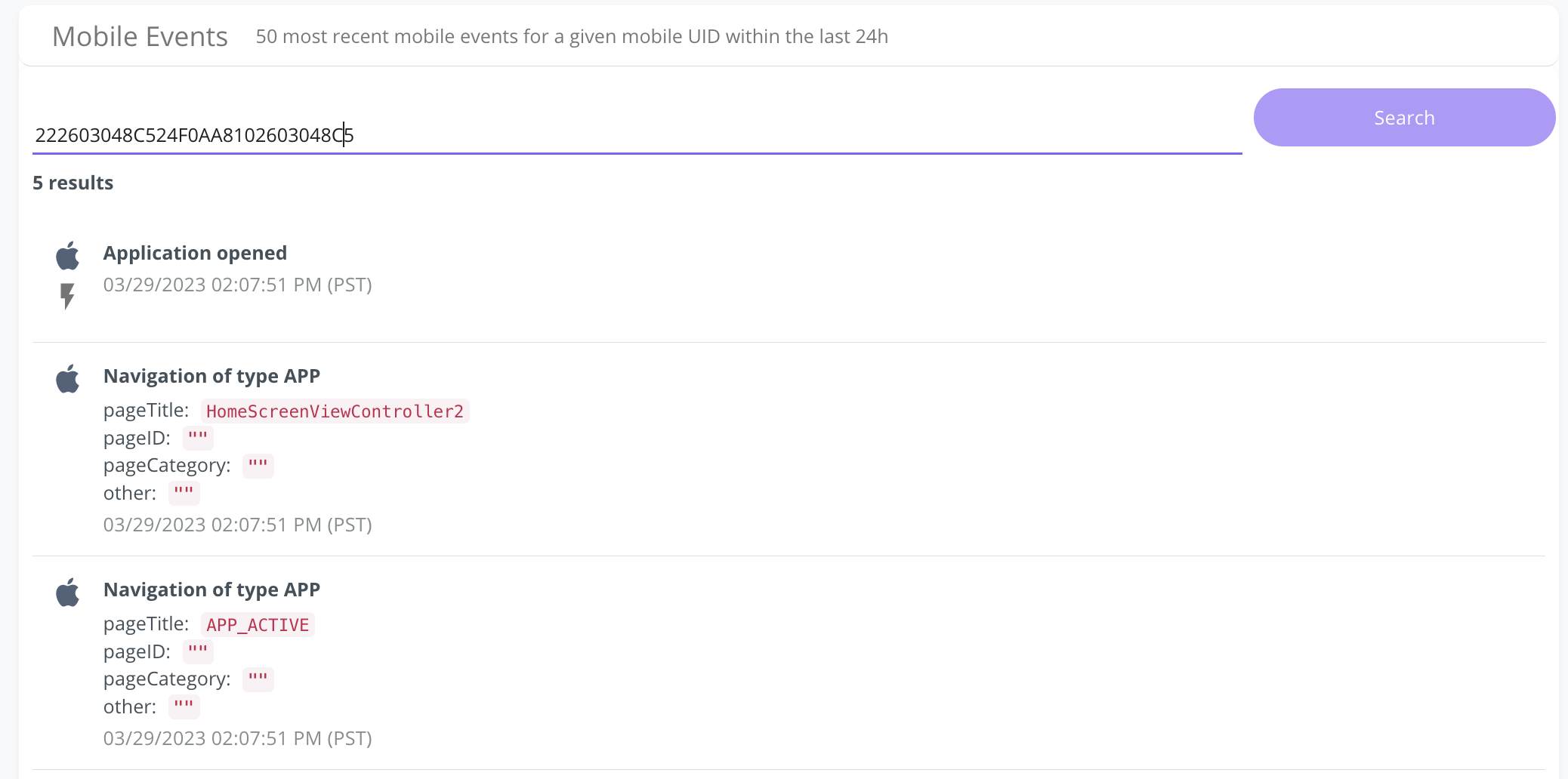
Step 4: Include mobileUID in backend and Forter API requests ✅
Send the latest token generated by the Forter SDKs to your backend when an order is placed via your native mobile application.
This will allow Forter to connect behavioral and cyber data collected on the mobile applications to the order data sent from your backend. Merchants are in charge of how the mobileUID is passed and most will pass it securely via API request headers. In the case of native mobile app orders, make sure that the following fields are populated:
- orderType: "MOBILE", "iOS", or "ANDROID" depending on your OS (mobile can be used for either OS)
- forterMobileUID: This should be populated instead of the
forterTokenCookie
if your app is fully native including your checkout page. If you use a webview for the checkout page, please review our Webview Integration. - mobileAppVersion: should reflect the version of the app the user is on
Example iOS Request
{
"orderId": "Example_order_fdse0rr489",
"orderType": "iOS",
"timeSentToForter": 1415287568000,
"checkoutTime": 1415273168,
"connectionInformation": {
"customerIP": "107.57.208.5",
"userAgent": "testStore 18.4.1 rv:184100001 (iPhone; iOS 12.4.1; en_US; iPhone10,3)",
"forterMobileUID": "6A9798AG16FF41F7B6B9878DD488ADD5_c2Vzc2lvbnRva2VuaGVyZQ==_CF4_ES4",
"mobileAppVersion": "18.4.1"
},
"totalAmount": {
"amountUSD": "99.95"
}
...
}
For SDK version 3.0.0 and above:
- The value returned from the Forter token listener callback should be used as the
forterMobileUID
.
For SDK versions prior to 3.0.0:
- The value passed to ForterSDK's
setDeviceUniqueIdentifier
method should be used as theforterMobileUID
.
Step 5: Deploy to Production 🚀
Merchants are in complete control of how and when they deploy Forter's SDK into their production environment. You'll work closely with your implementation team to ensure a seamless deployment of our SDK within your mobile app versions.
Updated 3 months ago