Android SDK
Step 1: Add to Maven
- If the Gradle version is 7.0 and above:
Inside your app'ssettings.gradle
, append the following repository, provided below, to your dependencyResolutionManagement block.
dependencyResolutionManagement {
repositoriesMode.set(RepositoriesMode.FAIL_ON_PROJECT_REPOS)
repositories {
google()
mavenCentral()
...
maven {
url "https://mobile-sdks.forter.com/android"
credentials {
username "<username-provided-by-forter>"
password "<password-provided-by-forter>"
}
}
}
}
- If the Gradle version is earlier than 7.0:
Inside your app'sbuild.gradle
, append the following repository, provided below, to your repositories block.
repositories {
...
maven { url 'https://maven.google.com' }
maven {
url "https://mobile-sdks.forter.com/android"
credentials {
username "<username-provided-by-forter>"
password "<password-provided-by-forter>"
}
}
}
NOTE: Specify and commit the credentials to your Git. Note that these credentials are not sensitive, but we keep them private to prevent bots and search engines from accessing the repository.
Step 2: Add the Forter3DS SDK dependency
Add the Forter3DS SDK as a dependency to your app's build.gradle
file under the dependencies block. Use the dependency provided below.
implementation 'com.forter.mobile:forter3ds:2.0.4'
implementation("com.forter.mobile:forter3ds:2.0.1")
Step 3: Manifest Permissions
Additionally, the Forter3DS
SDK requires the common permission: Internet. This permission is typically required by many applications and components. If you have not already included it in your permissions list, please add to your manifest file.
<!-- Forter3DS SDK permissions -->
<uses-permission android:name="android.permission.INTERNET" />
Initializing the SDK
The init
method is utilized to configure and set up the SDK, ensuring its readiness to handle transactions effectively
The SDK must be initialized from the main Application Context. If your app is not currently using an Application class, please add one and register it in the manifest.
Signature
fun init(
context: Context,
config: Forter3DSConfig,
callback: IForter3DSInitCallback?
)
Parameters
- context: An instance of the Android
Context
class, typically the application context. - config: An instance of
Forter3DSConfig
containing configuration parameters for the SDK initialization. - callback: An optional callback of type
IForter3DSInitCallback
to receive initialization status notifications.
Forter3DSConfig class
The Forter3DSConfig
class encapsulates configuration parameters required for initializing the SDK. It includes the following parameters:
- merchantId: Represents the unique identifier assigned to the merchant within the application. It typically aligns with the site ID unless a PSP necessitates distinct IDs per merchant.
- siteId: The site ID associated with the merchant account.
- defaultCustomization: Customization options for the default theme.
- darkCustomization: Customization options for the dark theme.
- monochromeCustomization: Customization options for the monochrome theme.
- shouldLoadTestServers: A boolean flag indicating whether to load test servers during initialization. Should be enabled only on testing environment.
IForter3DSInitCallback Interface
The IForter3DSInitCallback
interface defines callback methods to notify the client application of the initialization status. It includes the following methods:
- onInitializationSucceeded(): Invoked when the SDK initialization is successful.
- onInitializationFailed(): Invoked when the SDK initialization fails.
Usage
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.filters);
// Initialize Forter3DS
String mobileUID = Forter3DS.getInstance().generateDeviceUID(context);
Forter3DSConfig config = new Forter3DSConfig.Builder()
.setMerchantId(mobileUID)
.setSiteId("site_id")
.build();
Forter3DS.getInstance().init(this, config, new IForter3DSInitCallback() {
@Override
public void onInitializationSucceeded() {
// Handle success
}
@Override
public void onInitializationFailed() {
// Handle failure
}
});
}
val context: Context = applicationContext
val config = Forter3DSConfig.Builder()
.setMerchantId("your_merchant_id")
.setSiteId("your_site_id")
if (BuildConfig.DEBUG) {
config.loadTestServers()
}
Forter3DS.getInstance().init(context, config.build(), object : IForter3DSInitCallback {
override fun onInitializationSucceeded() {
// Handle successful initialization
}
override fun onInitializationFailed() {
// Handle initialization failure
}
})
FTR3DSCustomization class
The FTR3DSCustomization
class provides customization options for the UI elements in the native challenge screen. It allows developers to customize buttons, labels, text boxes, and toolbars to match the design and branding requirements of their application.
- setButtonCustomization: Customizes background color, corner radius and text color.
- setLabelCustomization: Customizes font size, text color and font.
- setTextBoxCustomization: Customizes border color, border width and corner radius.
- setToolbarCustomization: Customizes background color, button text and header text.
To create an instance of FTR3DSCustomization
, simply use its default constructor and apply the required customizations:
FTR3DSCustomization defaultCustomization = new FTR3DSCustomization();
FTR3DSButtonCustomization button = new FTR3DSButtonCustomization();
button.setBackgroundColor("#ebe134");
defaultCustomization.setButtonCustomization(button, FTR3DSButtonType.SUBMIT);
val defaultCustomization = FTR3DSCustomization().apply {
val button = FTR3DSButtonCustomization().apply {
setBackgroundColor("#ebe134")
}
setButtonCustomization(button, FTR3DSButtonType.SUBMIT)
}
Set the FTR3DSCustomization to Forter3DSConfig
Forter3DSConfig config = new Forter3DSConfig.Builder()
.setDefaultCustomization(defaultCustomization)
.setMerchantId(mobileUID)
.setSiteId(siteId)
.loadTestServers()
.build();
val config = Forter3DSConfig.Builder()
.setDefaultCustomization(defaultCustomization)
.setMerchantId(mobileUID)
.setSiteId(siteId)
.loadTestServers()
.build()
Create Transaction
Upon completion of the Init stage, Forter can collect the necessary data to create an FTRTransaction object.
To create the object, utilize the createTransaction method to create an FTRTransaction object which contains the data required for the server in order to create a transaction.
Arguments
- dsId: the specific directory identifier, retrieved from the init call made to the server.
- version: 3DS version, retrieved from the init call made to the server.
Usage
- In 3DS1 flow - the directory server identifier is not required
- In 3DS2 flow - both parameters are required
Return Value
createTransaction method returns a FTRTransaction object which contains the properties seen on the right.
These parameters are required for creating a challenge in 3DS2 flows if needed. In the case of 3DS1, all the fields will be null.
The following parameters should be used to create the threeDSMobileAppSDKData object in Adaptive Auth Transaction API call, which is usually called from the server:
- sdkEncData: A string that represents the encrypted device data.
- sdkAppID: The 3DS SDK uses a secure random function to generate the App ID in UUID format. This ID is unique and is generated during installation and update of the app.
- sdkEphemeralPubKey: Returns the SDK Ephemeral Public Key. For each transaction the createTransaction method will generate a fresh ephemeral key pair and this property will hold the public key component as a String representation.
- sdkTransID: Returns the SDK transaction ID. For each transaction the createTransaction method will generate a transaction ID in UUID format.
- sdkReferenceNumber: A string that represents the SDK reference number.
The 3DS server expects these parameters to be encoded with base64. To convert this object to the required format, use toBase64 method.
NOTE: When making the doChallenge call, the acsTransId, threeDSServerTransId, and version parameters are mandatory (if this is the required flow). It's crucial to pass these parameters "as is" without any parsing when calling the doChallenge method.
While it's recommended to get these parameters from your server before calling doChallenge, it's advised not to store them locally. This approach helps ensure that the most up-to-date parameters are used in the doChallenge call.
Do Challenge
If Forter's decision is to decline the transaction and a verification is required, a challenge flow must be initiated. To do so, the app should call the doChallenge method.
Parameters
The doChallenge method requires the parameters provided below, with the mandatory params for each type marked with ✅ and optional values marked with '?'.
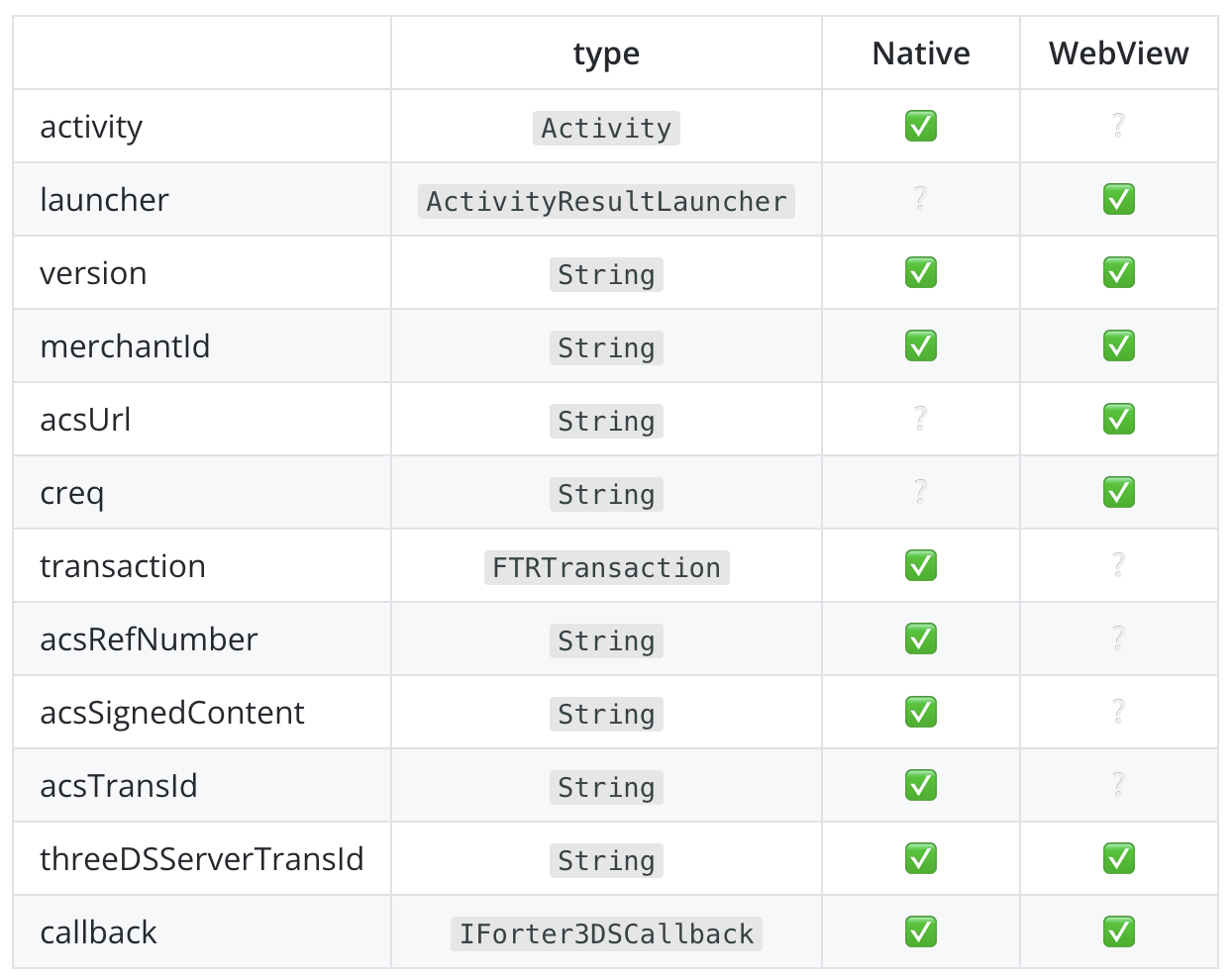
Notes:
- activity argument passed is the Android activity instance that invoked doChallenge
- launcher argument passed is a callback for an activity result
- callback argument passed is the object that will implement the IForter3DSCallback interface callback methods
Return Value
doChallenge method does not return any value
Callbacks
Callback object must be implemented for notifying the app about the challenge status:
- onChallengeFinished: This callback is triggered when the challenge is successfully completed.
- onChallengeFail: This callback is triggered when the challenge fails.
- onChallengeSkipped: This callback is triggered when a challenge is not required.
val challengeParams = FTR3DSChallengeParams.Builder(
"2.1.0",
"7a845fd7-9b2f-4849-9e12-a0e578301612"
)
.setMerchantId("a1-b2-c3-d4")
.setAcsRefNumber("nds-internal-acs-approval")
.setAcsSignedContent("eyJhbGc...")
.setAcsTransId("e1a1a0a5-29d9-4602-b421-dca64c69df57")
.build()
Forter3DS.getInstance().doChallenge(
this, challengeLauncher,
transaction, challengeParams, this
)
)
Updated about 2 months ago